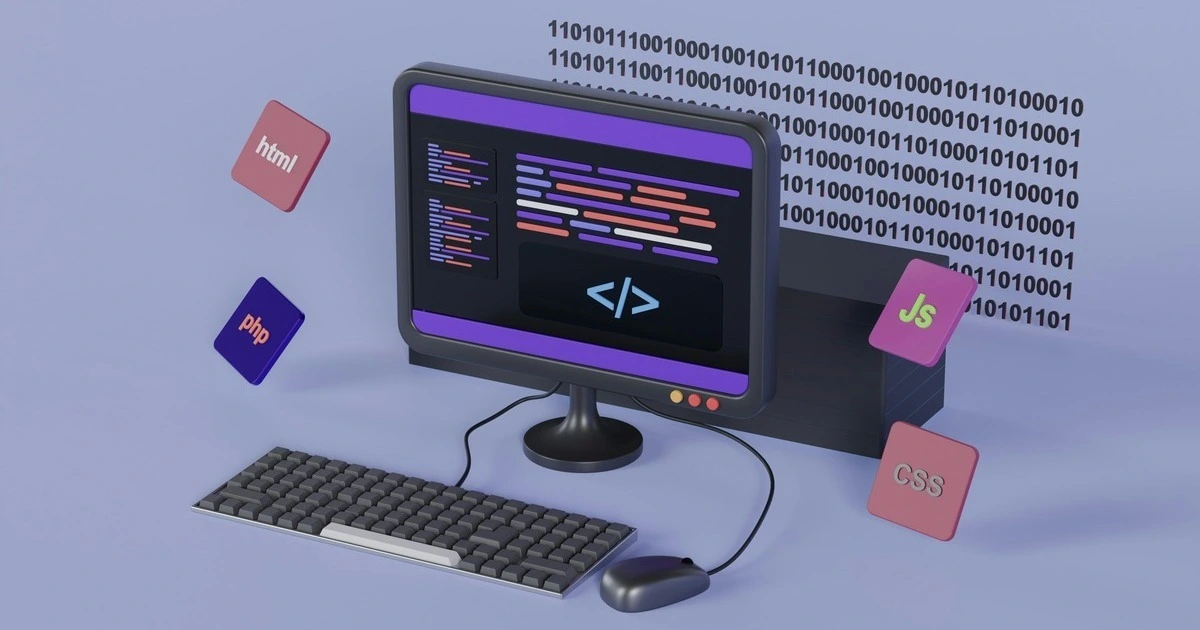
Photo by Growtika on Unsplash
What is Webpack?
Alright, let’s talk about Webpack—because chances are, you’ve heard of it but might still be wondering what exactly it does. Simply put, Webpack is a tool that takes all your JavaScript, CSS, images, and other assets and bundles them together so your website loads faster and runs smoother.
Imagine you’re packing for a trip. Instead of carrying 10 different bags, wouldn’t it be easier to fit everything into one organized suitcase? That’s exactly what Webpack does for your code!
Why Do We Need Webpack?
The Problem Before Webpack
Back in the day, websites loaded multiple JavaScript files and CSS stylesheets separately. This led to:
- Slow load times (more files = more requests = longer waiting).
- Messy dependency management (files relying on each other could break easily).
- Bloated, unoptimized code (unnecessary scripts and styles slowing things down).
The Webpack Solution
Webpack makes life easier by:
- Combining all files into a single, optimized bundle.
- Minimizing and compressing code to improve performance.
- Handling modern JavaScript (ES6+), TypeScript, and CSS preprocessors effortlessly.
- Improving page speed by eliminating unused code (tree shaking) and loading only what's needed (lazy loading).
- Providing a development server that auto-refreshes when changes are made. Basically, it takes all the annoying, repetitive parts of frontend development and automates them.
How Does Webpack Work?
At its core, Webpack follows a simple process: Find, Process, Bundle.
1. Entry Point
Webpack starts by looking at the main JavaScript file—the entry point—to figure out what the project needs.
module.exports = { entry: './src/index.js', };
2. Output
Once Webpack processes everything, it spits out the final bundled file.
module.exports = { output: { filename: 'bundle.js', path: __dirname + '/dist', }, };
3. Loaders
Loaders help Webpack understand files beyond JavaScript, like CSS and images.
module.exports = { module: { rules: [ { test: /\.css$/, use: ['style-loader', 'css-loader'], }, ], }, };
4. Plugins
Plugins supercharge Webpack by adding extra features, like generating an HTML file automatically.
const HtmlWebpackPlugin = require('html-webpack-plugin'); module.exports = { plugins: [ new HtmlWebpackPlugin({ template: './src/index.html' }), ], };
5. Development vs. Production Mode
Webpack has different modes for development and production. Development mode gives fast builds, while production mode optimizes everything for the best performance.
module.exports = { mode: 'development', // or 'production' };
Wrapping Up
Webpack is like that friend who organizes everything so I don’t have to. It takes multiple files, optimizes them, and ensures the site loads fast. Whether you’re working with JavaScript, CSS, images, or even TypeScript, Webpack has your back.