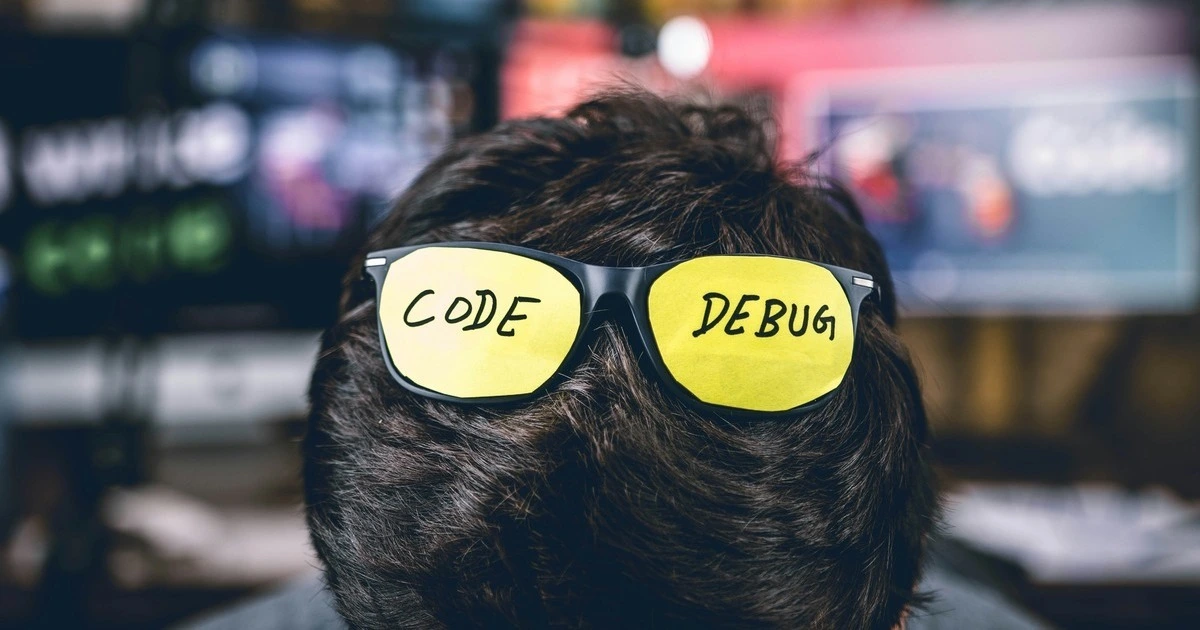
Photo by Hitesh Choudhary on Unsplash
Unlocking the Power of the Console Object: Beyond console.log()
The console
object is like the Swiss Army knife of debugging—except most developers only use one tool: console.log()
. But what if I told you there’s an entire world of powerful methods hidden inside console
, waiting to make your debugging experience 10x better? In this guide, we’ll explore the underrated and often ignored features of the console
object that will boost your JavaScript debugging skills.
1. console.table()
– Pretty Print Your Data 📊
Tired of messy arrays and objects cluttering your console? console.table()
makes them readable!
const users = [ { id: 1, name: 'Alice', role: 'Admin' }, { id: 2, name: 'Bob', role: 'User' }, { id: 3, name: 'Charlie', role: 'Editor' } ]; console.table(users);
This outputs a neat table instead of an unreadable object dump. Perfect for debugging API responses!
2. console.warn()
& console.error()
– Color Code Your Logs ⚠️
If all your logs look the same, debugging becomes a nightmare. Use console.warn()
for warnings and console.error()
for errors to make important messages stand out.
console.warn('This is a warning!'); // Yellow text console.error('Something went wrong!'); // Red text
Errors even include a stack trace, helping you track down issues faster!
3. console.group()
– Organize Logs Like a Pro 📂
Ever wanted to categorize logs into collapsible sections? console.group()
and console.groupEnd()
let you do just that!
console.group('User Info'); console.log('Name: Alice'); console.log('Role: Admin'); console.groupEnd();
Now, your console stays clean and structured. Nested groups? Also possible! 😎
4. console.count()
– Track Function Calls 🔢
Need to track how many times a function is executed? console.count()
keeps count for you.
function fetchData() { console.count('fetchData called'); // API call simulation } fetchData(); fetchData(); fetchData();
This prints:
fetchData called: 1
fetchData called: 2
fetchData called: 3
Super useful for debugging loops and recursive functions!
5. console.time()
– Measure Performance ⏱️
Performance optimization starts with measuring execution time. console.time()
and console.timeEnd()
make it easy.
console.time('Loop Timer'); for (let i = 0; i < 1000000; i++) { // Some heavy computation } console.timeEnd('Loop Timer');
Now you’ll know exactly how long your functions take to run. 🚀
6. console.trace()
– See the Call Stack 🔍
Need to debug function calls? console.trace()
prints a full call stack.
function first() { second(); } function second() { third(); } function third() { console.trace('Trace log'); } first();
Now you can see exactly where your function was called from!
7. console.assert()
– Conditional Debugging ✅
Instead of manually checking conditions, let console.assert()
do the work.
const age = 15; console.assert(age >= 18, 'User is not an adult!');
If the condition is false, an error message appears. Great for debugging validation logic!
8. console.dir()
– Inspect Objects in Depth 🔎
When console.log()
isn’t enough, console.dir()
provides a deeper look at object properties and methods.
console.dir(document.body);
This is especially useful for exploring DOM elements!
9. console.clear()
– Declutter Your Console 🧹
Want to start fresh? console.clear()
wipes your console clean.
console.clear();
Perfect when debugging gets messy!
Final Thoughts 🏁
The console
object is far more powerful than just console.log()
. By mastering these advanced methods, you can debug faster, write cleaner code, and look like a JavaScript genius in front of your teammates.